Sprite::setOnEdgeBounce()
Sets wether the sprite should bounce when hitting the edge or not.
Examples
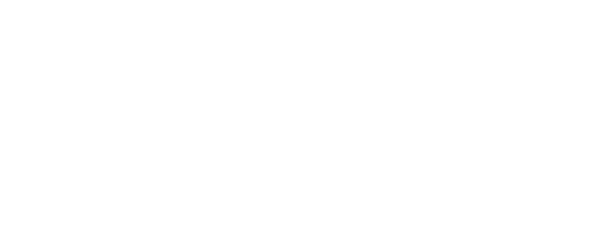
import org.openpatch.scratch.*;
public class SpriteSetOnEdgeBounce {
public SpriteSetOnEdgeBounce() {
Stage myStage = new Stage(600, 240);
Sprite mySprite = new Sprite("slime", "assets/slime.png");
myStage.add(mySprite);
mySprite.setOnEdgeBounce(true);
mySprite.setRotationStyle(RotationStyle.LEFT_RIGHT);
while (myStage.getTimer().forMillis(3000)) {
mySprite.move(1);
myStage.wait(20);
}
myStage.exit();
}
public static void main(String[] args) {
new SpriteSetOnEdgeBounce();
}
}
Syntax
Java
.setOnEdgeBounce(shouldBounce)
Scratch
if on edge, bounce
Parameters
Name | Data Type | Description |
---|---|---|
shouldBounce | boolean | If true the sprite will bounce when hitting the edge. |
Return
void