Sprite::lerp()
Calculates a number between the start and the stop by using the given amount.
Examples
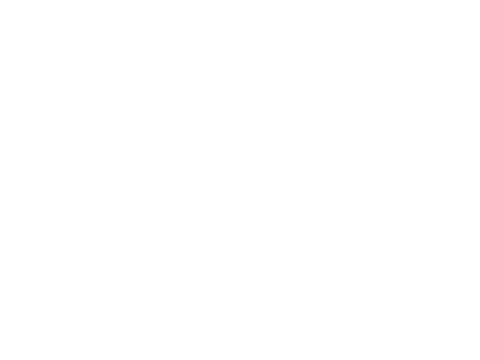
import org.openpatch.scratch.*;
import org.openpatch.scratch.extensions.pen.*;
import org.openpatch.scratch.extensions.timer.*;
public class OperatorsLerp {
public OperatorsLerp() {
Stage myStage = new Stage();
Pen myPen = new Pen();
myStage.add(myPen);
myPen.down();
int startMillis = Timer.millis();
for (int currentMillis = Timer.millis();
currentMillis - startMillis < 3000;
currentMillis = Timer.millis()) {
double x =
Operators.lerp(
0, Window.getInstance().getWidth(), (currentMillis - startMillis) / 3000.0);
double y =
Operators.lerp(
0, Window.getInstance().getHeight(), (currentMillis - startMillis) / 3000.0);
myPen.setPosition(x, y);
}
myStage.exit();
}
public static void main(String[] args) {
new OperatorsLerp();
}
}
Syntax
Java
.lerp(start, stop, amount)
Scratch
lerp (start) (stop) (amount)
Parameters
Name | Data Type | Description |
---|---|---|
amount | float | Changes the tint by the amount. |
Return
void