Sprite::setRotationStyle()
Sets the rotation style of the sprite. This influences how different rotations are visually expressed. You should use the enum RotationStyle for setting this value.
There are three options for you to choose. All around means the sprite can face any of the 360 degrees. It is the default. Left-right means the sprite can only face left or right, and any other directions are rounded. The sprite will also be horizontally flipped when facing left in the left-right style. Don't rotate means that the sprite always faces as in 0°.
Examples
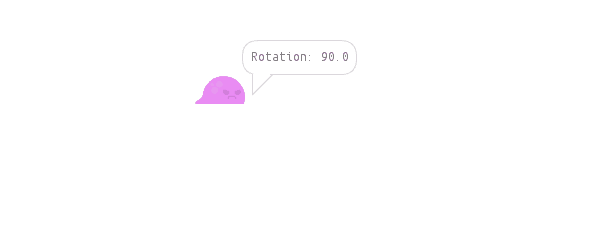
import org.openpatch.scratch.RotationStyle;
import org.openpatch.scratch.Sprite;
import org.openpatch.scratch.Stage;
public class SpriteSetRotationStyle {
public SpriteSetRotationStyle() {
Stage myStage = new Stage(600, 240);
Sprite mySprite = new Sprite("slime", "assets/slime.png");
myStage.add(mySprite);
mySprite.changeX(-80);
mySprite.changeY(30);
mySprite.say("Rotation: " + mySprite.getDirection());
myStage.wait(1000);
mySprite.setDirection(45);
mySprite.say("All-Around: " + mySprite.getDirection());
myStage.wait(1000);
mySprite.setRotationStyle(RotationStyle.DONT);
mySprite.setDirection(180);
mySprite.say("Don't: " + mySprite.getDirection());
myStage.wait(1000);
mySprite.setRotationStyle(RotationStyle.LEFT_RIGHT);
mySprite.setDirection(200);
mySprite.say("LEFT-RIGHT: " + mySprite.getDirection());
myStage.wait(1000);
myStage.exit();
}
public static void main(String[] args) {
new SpriteSetRotationStyle();
}
}
Syntax
Java
.setRotationStyle(style)
Scratch
set rotation style [ v]
Parameters
Name | Data Type | Description |
---|---|---|
style | RotationStyle | Sets the rotation style. |
Return
void