AnimatedSprite::playAnimation()
Plays an animation.
Examples
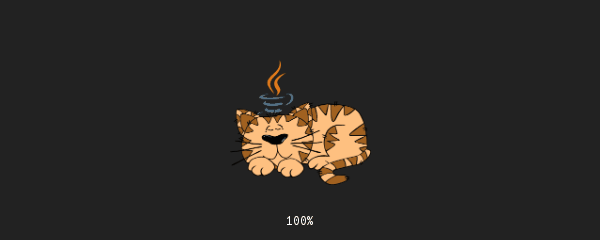
import org.openpatch.scratch.extensions.animation.AnimatedSprite;
public class MySprite extends AnimatedSprite {
public MySprite() {
this.addAnimation("idle", "assets/bee_idle.png", 6, 36, 34);
}
public void run() {
this.playAnimation("idle");
}
}
import org.openpatch.scratch.Stage;
public class MyStage extends Stage {
public MyStage() {
this.add(new MySprite());
}
}
import org.openpatch.scratch.Stage;
import org.openpatch.scratch.Window;
public class MyWindow extends Window {
public MyWindow() {
Stage myStage = new MyStage();
this.setStage(myStage);
// Wait for 5 seconds
while (myStage.getTimer().forMillis(5000))
;
this.exit();
}
public static void main(String[] args) {
new MyWindow();
}
}
Syntax
Java
.playAnimation(name)
.playAnimation(name, once)
Scratch
play animation (name)
Parameters
Name | Data Type | Description |
---|---|---|
name | String | The name of the animation to play |
once | boolean | If true plays the animation only once. |
Return
void