Timer::intervalMillis()
Returns true for the first interval and then false for the second interval. The starting interval can bechanged by passing a true as a third parameter.
Examples
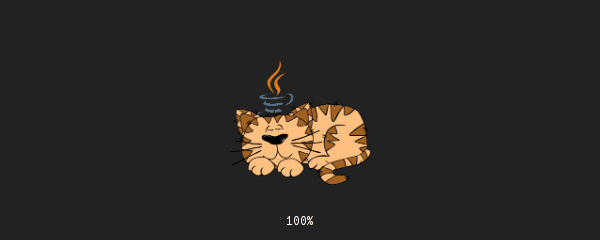
import org.openpatch.scratch.*;
import org.openpatch.scratch.extensions.timer.Timer;
public class TimerIntervalMillis {
public TimerIntervalMillis() {
Stage myStage = new Stage(600, 240);
Timer myTimer = new Timer();
while (myStage.getTimer().forMillis(3000)) {
if (myTimer.intervalMillis(500)) {
myStage.display("Interval 1");
} else {
myStage.display("Interval 2");
}
}
myStage.exit();
}
public static void main(String[] args) {
new TimerIntervalMillis();
}
}
import org.openpatch.scratch.*;
import org.openpatch.scratch.extensions.timer.Timer;
public class TimerIntervalMillis2 {
public TimerIntervalMillis2() {
Stage myStage = new Stage(600, 240);
Timer myTimer = new Timer();
while (myStage.getTimer().forMillis(3000)) {
if (myTimer.intervalMillis(500, 1000)) {
myStage.display("Interval 1");
} else {
myStage.display("Interval 2");
}
}
myStage.exit();
}
public static void main(String[] args) {
new TimerIntervalMillis2();
}
}
import org.openpatch.scratch.*;
import org.openpatch.scratch.extensions.timer.Timer;
public class TimerIntervalMillis3 {
public TimerIntervalMillis3() {
Stage myStage = new Stage(600, 240);
Timer myTimer = new Timer();
while (myStage.getTimer().forMillis(3000)) {
if (myTimer.intervalMillis(500, true)) {
myStage.display("Interval 1");
} else {
myStage.display("Interval 2");
}
}
myStage.exit();
}
public static void main(String[] args) {
new TimerIntervalMillis3();
}
}
Syntax
Java
.intervalMillis(millis1)
.intervalMillis(millis1, millis2)
.intervalMillis(millis, true)
.intervalMillis(millis1, millis2, true)
Scratch
interval () () <> millis :: boolean
Parameters
Name | Data Type | Description |
---|---|---|
millis1 | boolean | Time in milliseconds for the first interval |
millis2 | boolean | Time in milliseconds for the seconds interval |
skipFirst | boolean | If true start with the second interval |
Return
boolean