AnimatedSprite::setAnimationInterval()
Sets the animation interval. The animation interval determines how fast an animation is played. Lower numbers mean faster playback.
Examples
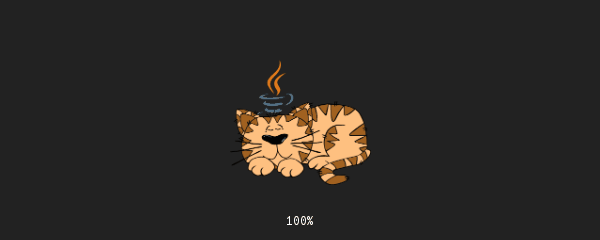
import org.openpatch.scratch.*;
import org.openpatch.scratch.extensions.animation.*;
public class AnimatedSpriteSetAnimationInterval {
public AnimatedSpriteSetAnimationInterval() {
Stage myStage = new Stage(600, 240);
AnimatedSprite bee = new AnimatedSprite();
bee.addAnimation("idle", "assets/bee_idle.png", 6, 36, 34);
myStage.add(bee);
bee.changeY(30);
while (myStage.getTimer().forMillis(2000)) {
bee.playAnimation("idle");
bee.say("Interval: " + bee.getAnimationInterval());
}
bee.setAnimationInterval(20);
myStage.getTimer().reset();
while (myStage.getTimer().forMillis(2000)) {
bee.playAnimation("idle");
bee.say("Interval: " + bee.getAnimationInterval());
}
myStage.exit();
}
public static void main(String[] args) {
new AnimatedSpriteSetAnimationInterval();
}
}
Syntax
Java
.setAnimationInterval(interval)
Scratch
Parameters
Name | Data Type | Description |
---|---|---|
interval | int | Animation interval. Lower numbers mean faster playback. |
Return
void