AnimatedSprite::resetAnimation()
Resets an animation. This is useful for example, when an animation should only be played once, but maybe on a user interaction should play again.
Examples
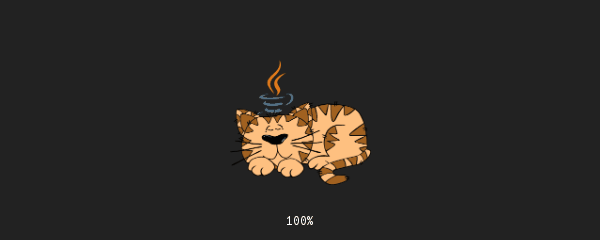
import org.openpatch.scratch.KeyCode;
import org.openpatch.scratch.extensions.animation.AnimatedSprite;
public class MySprite extends AnimatedSprite {
public MySprite() {
this.addAnimation("idle", "assets/bee_idle.png", 6, 36, 34);
}
public void run() {
this.playAnimation("idle");
if (this.isKeyPressed(KeyCode.VK_SPACE)) {
this.resetAnimation();
}
}
}
import org.openpatch.scratch.Stage;
public class MyStage extends Stage {
public MyStage() {
this.add(new MySprite());
}
}
import org.openpatch.scratch.Stage;
import org.openpatch.scratch.Window;
public class MyWindow extends Window {
public MyWindow() {
Stage myStage = new MyStage();
this.setStage(myStage);
// Wait for 5 seconds
while (myStage.getTimer().forMillis(5000))
;
this.exit();
}
public static void main(String[] args) {
new MyWindow();
}
}
Syntax
Java
.resetAnimation()
Scratch
reset animation
Return
void